일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- jwttoken
- 개발자부트캠프추천
- @FeignClient
- 구글 OAuth login
- KPT회고
- 커스텀 헤더
- Python
- 파이썬
- 프로그래머스
- jwt
- Spring multimodule
- 취업리부트코스
- 1주일회고
- JavaScript
- 코딩테스트 준비
- 99클럽
- 단기개발자코스
- 항해99
- 개발자 취업
- infcon 2024
- 인프콘 2024
- DesignPattern
- 디자인패턴
- 프로그래머스 이중우선순위큐
- TiL
- 빈 조회 2개 이상
- spring batch 5.0
- 빈 충돌
- 전략패턴 #StrategyPattern #디자인패턴
- 디자인 패턴
- Today
- Total
목록분류 전체보기 (192)
m1ndy5's coding blog
https://leetcode.com/problems/permutations/ class Solution: def permute(self, nums: List[int]) -> List[List[int]]: answer = [] def dfs(pair, n): if len(pair) == n: answer.append(pair[:]) return for i in nums: if i not in pair: pair.append(i) dfs(pair, n) pair.pop() dfs([], len(nums)) return answer간단하게 라이브러리 사용해서 풀기 import itertools def permute(self, nums): return list(itertools.permutations(nums..
https://leetcode.com/problems/letter-combinations-of-a-phone-number/ class Solution: def letterCombinations(self, digits: str) -> List[str]: dic = {'2': ['a', 'b', 'c'], '3': ['d', 'e', 'f'], '4': ['g', 'h', 'i'], '5': ['j', 'k', 'l'], '6': ['m', 'n&..
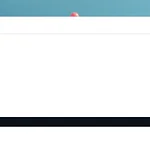
항해99 단기 개발자 취업 코스에 합류하게 되어 1월 3일부터 진행하고 있다. 알고리즘 공부도 하고 남는 시간엔 스프링 인강도 들으면서 공부하고 있다! 드디어 백준 골드 찍었다!! 하하 늦은감이 없진 않지만 이제부터 올리면 되는거 아니겠냐구??ㅎㅎㅎㅎ 그리고 스프링 MVC 1편도 다들었다!! 하하 이것도 늦은감이 없진 않지만 또 앞으로 열심히 들으면 되는 거 아니겠냐구~~ 어쨌든 2023년에 미뤄놨던 일들을 새해 초에 달성하게 되어서 의욕이 더 생겨나는 주였던 것 같다. 다음주부터는 1. 스프링 MVC 2편 인강 듣기 2. 프로젝트 진행하기 3. 알고리즘 수업 따라가기 가 내 목표다! 화이팅 뽜이아~
https://leetcode.com/problems/odd-even-linked-list/ # Definition for singly-linked list. # class ListNode: # def __init__(self, val=0, next=None): # self.val = val # self.next = next class Solution: def oddEvenList(self, head: Optional[ListNode]) -> Optional[ListNode]: # 아무것도 들어오지 않았을 때 if head is None: return None odd = head even = head.next even_head = head.next # 짝수번째도 있고 그 다음 홀수번째도 있을 때 whil..
https://leetcode.com/problems/merge-two-sorted-lists/ # Definition for singly-linked list. # class ListNode: # def __init__(self, val=0, next=None): # self.val = val # self.next = next class Solution: def mergeTwoLists(self, list1: Optional[ListNode], list2: Optional[ListNode]) -> Optional[ListNode]: # 새로운 리스트노드 생성 curr = dummy = ListNode() while list1 and list2: # 더 작은 값을 현재 뒤에다 붙임 if list1.val..
https://leetcode.com/problems/reverse-linked-list/ # Definition for singly-linked list. # class ListNode: # def __init__(self, val=0, next=None): # self.val = val # self.next = next class Solution: def reverseList(self, head: Optional[ListNode]) -> Optional[ListNode]: node, prev = head, None while node: next, node.next = node.next, prev prev, node = node, next return prev 연결리스트를 거꾸로 뒤집는 문제다 이 때 ..
https://leetcode.com/problems/kth-largest-element-in-an-array/ import heapq class Solution: def findKthLargest(self, nums: List[int], k: int) -> int: nums = [-1 * num for num in nums] heapq.heapify(nums) answer = 0 for _ in range(k): answer = heapq.heappop(nums) return answer * -1최대힙처럼 작동해야했기 때문에 -1을 곱했다. sort로 풀어도 문제없는 문제였다.
https://leetcode.com/problems/the-k-weakest-rows-in-a-matrix/ import heapq class Solution: def kWeakestRows(self, mat: List[List[int]], k: int) -> List[int]: order = [row.count(1) for row in mat] heap = order[:] heapq.heapify(heap) visited = [0 for _ in range(len(order))] answer = [] for _ in range(k): m = heapq.heappop(heap) for i, v in enumerate(order): if v == m and visited[i] == 0: answer.ap..
https://leetcode.com/problems/maximum-product-of-two-elements-in-an-array/ python heapq는 기본적으로 minheap구조로 되어있다. 따라서 max값을 구하고 싶을 때는 -1을 곱해서 힙에 넣고 최솟값을 뺀 뒤 다시 -1을 곱해주면 된다. import heapq class Solution: def maxProduct(self, nums: List[int]) -> int: nums = [-1 * num for num in nums] heapq.heapify(nums) # -1를 곱했기 때문에 1을 빼려면 1을 더해주면 된다 혹은 리턴할 때 1을 빼고 곱해주거나! a = heapq.heappop(nums)+1 b = heapq.heappop(..
https://leetcode.com/problems/top-k-frequent-elements/ class Solution: def topKFrequent(self, nums: List[int], k: int) -> List[int]: dic = {} answer = [] for num in nums: if num not in dic: dic[num] = 1 else: dic[num] += 1 #[[1, 3번], [2, 2번], ...] l = [[key, value] for key, value in dic.items()] # 빈도수 기준 정렬(내림차순) l.sort(key=lambda x: x[1], reverse = True) # k개만 뽑기 for i in range(k): answer.appen..